Variables: The Containers of Spatial Information
Variables serve as placeholders to store different types of data, and in a GIS environment, these can encompass geographic coordinates, attribute values, and more. PHP variables are declared using the `$` symbol, followed by a variable name. For instance:
$latitude = 34.0522;
$longitude = -118.2437;
$cityName = "Los Angeles";
In this example, we've assigned values to variables representing latitude, longitude, and the name of a city.
Data Types: Crafting a Spatial Data Palette
PHP supports various data types, each tailored to handle specific kinds of information. In a GIS context, you'll frequently encounter:
1. Numbers (integers and floats): These handle numeric values like coordinates, distances, or measurements.
2. Strings: These hold text information such as names of cities, countries, or geographic features.
3. Arrays: Arrays can store multiple values, making them ideal for holding lists of coordinates or attribute data.
4. Boolean: Boolean values (`true` or `false`) can be employed to represent conditions or spatial relationships.
Operators: Navigating the GIS Logic
Operators are the tools that enable you to manipulate and combine data in meaningful ways. In a GIS-centric PHP script, you'll frequently use:
1. Arithmetic Operators: These help perform mathematical calculations, which can be useful for distance calculations, scaling data, or area measurements.
2. String Operators: Concatenation (`.`) allows you to combine strings, which is handy for constructing dynamic labels or descriptions for GIS features.
3. Comparison Operators: These let you compare values, facilitating spatial analysis by evaluating conditions like distance relationships or attribute comparisons.
4. Logical Operators: These are essential for constructing GIS decision logic. For example, you can use logical operators to filter and process data based on specific conditions.
Putting It All Together: A Simple GIS Example
Imagine you're building a web application that calculates the distance between two geographic points. You could use variables to store the latitude and longitude of these points, employ arithmetic operators to compute the distance, and use string operators to craft a meaningful output
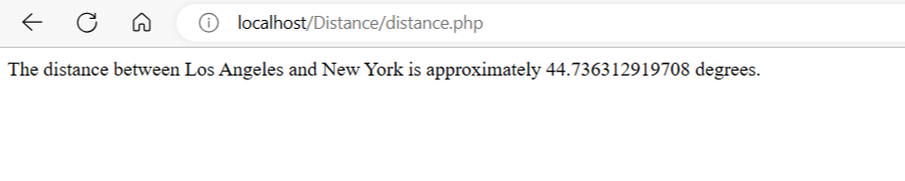
$distance = sqrt(pow($lat2 - $lat1, 2) + pow($lon2 - $lon1, 2));
Explanation:
1. `$lat1` and `$lon1`: These are variables that store the latitude and longitude of one point.
2. `$lat2` and `$lon2`: These are variables that store the latitude and longitude of another point.
3. `pow($lat2 - $lat1, 2)`: This part calculates the squared difference between the latitudes of the two points. It subtracts the latitude of the first point from the latitude of the second point and then raises the result to the power of 2.
4. `pow($lon2 - $lon1, 2)`: Similarly, this part calculates the squared difference between the longitudes of the two points.
5. Adding the squared latitude and squared longitude differences: The squared differences in latitude and longitude are added together.
6. `sqrt(...)`: Finally, the square root of the sum of the squared differences is calculated.
Overall Purpose:
The code is used to calculate the distance between two points in a two-dimensional space. The points are defined by their latitudes and longitudes. It uses the Pythagorean theorem, which you might have learned in geometry class. The theorem calculates the distance between two points as if you were drawing a straight line between them.
In this code, the squared differences in latitudes and longitudes are calculated separately and then added together. Taking the square root of this sum gives you the actual distance between the two points. This approach works well for small distances on a flat surface, but for larger distances or on a curved surface like Earth, more complex formulas would be needed to account for the curvature of the planet.
So, this line of code essentially computes the straight-line distance between two points given their latitude and longitude coordinates.
`$distanceDegrees` is the decimal distance in degrees that you want to convert to kilometers. You can replace it with the actual distance you have.
`$kmPerDegree` is an approximate conversion factor that represents the average distance in kilometers covered by one degree of latitude. It's based on the Earth's average radius.
The `$distanceKilometers` variable holds the result of the conversion by multiplying the decimal distance by the conversion factor.
Finally, the `echo` statement displays the converted distance in kilometers.
Here is the result:-
Variables, data types, and operators form the foundation of any PHP script, especially when it comes to GIS applications. Whether you're calculating distances, analyzing spatial relationships, or visualizing geographic data, a solid understanding of these concepts is essential. By mastering these fundamentals, you're not only opening the door to creating dynamic and interactive GIS applications but also laying the groundwork for more advanced spatial programming endeavors. As you continue your journey, remember that the fusion of PHP and GIS has the potential to unlock a new dimension of geospatial exploration and understanding.